Understanding Coroutines and Tasks in Python's Asyncio Library
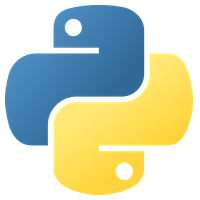
This section provides an overview of coroutines and tasks in Python's asyncio library, emphasizing their use in asynchronous programming. Coroutines, defined using the async/await
syntax, allow for non-blocking operations, such as sleeping for a second while other tasks can run concurrently. The document explains how to create and run coroutines using functions like asyncio.run()
and asyncio.create_task()
. It introduces the TaskGroup
class for managing multiple tasks together and highlights various awaitable objects, including Tasks
and Futures
, as well as methods for handling timeouts and cancellations. Additionally, it touches upon running tasks in threads and introspection features for tasks.
- Coroutines are defined with
async def
and can be awaited. asyncio.create_task()
schedules coroutines to run concurrently.TaskGroup
manages related tasks, ensuring they complete together.- Tasks can be cancelled, and
asyncio.timeout()
is used to limit wait times. - The library supports running I/O-bound functions in separate threads using
asyncio.to_thread()
.
What is a coroutine in asyncio?
Coroutines are special functions defined with async def
that can be paused and resumed during execution, allowing other tasks to run in the meantime.
How do I create a task in asyncio?
You can create a task by calling asyncio.create_task(coro)
, which schedules a coroutine to run concurrently with other tasks.
What is the purpose of TaskGroup
in asyncio?
TaskGroup
is a context manager that allows you to manage multiple tasks together, waiting for all to complete and handling exceptions in a structured way.